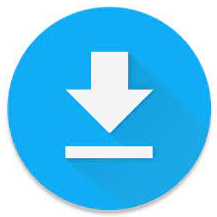
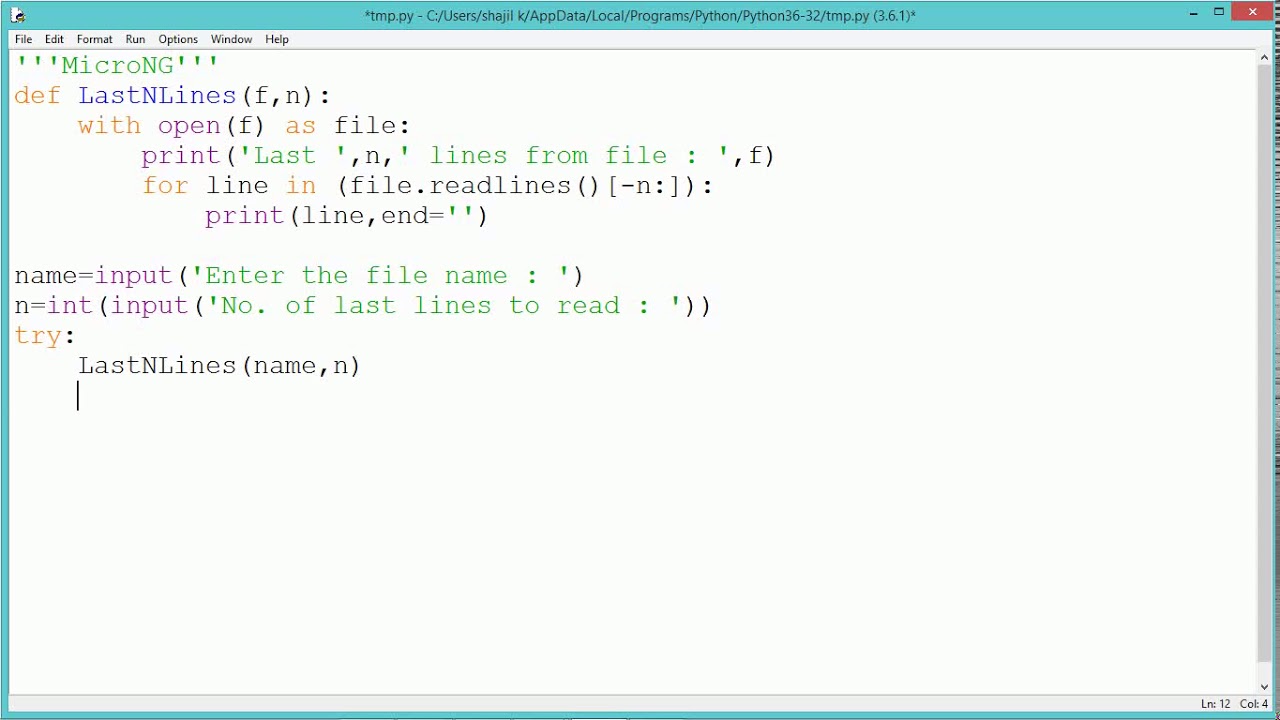
By using Python’s with open(), the opened file resource will only be available in the indented block of code:

Therefore, with modern Python, it’s recommended to use the with-statement when you can. And this is a trivial example, but you can imagine that with more complicated software, in which exceptions might get caught so the software can keep running, it can go wrong quickly.Īnother problem that may arise, is that you simply forget to write the close() call. If an exception occurs while Python reads the file, the f.close() call will never be reached. There’s a potential problem in the previous example. This could be a problem with large files, but we’ll get to that soon. This is no problem for small files, but do realize that you load all the contents into memory at once. If you want to read all the file content into a single string, at once, use the read() method on a file object, without arguments. In the example above, you can see how we read all contents from the file so we can print it. If the interactive example above doesn’t work for some reason, here’s a text version of the same code: f = open('text.txt')į.close() Python read file content at once It should work the same Python won’t complain and will close the file resource on exit. If you’re feeling adventurous, try and remove the f.close(). You should prefer using the with-statement. To illustrate why the with statement is so useful, let’s first open and close a file in the traditional, manual way:
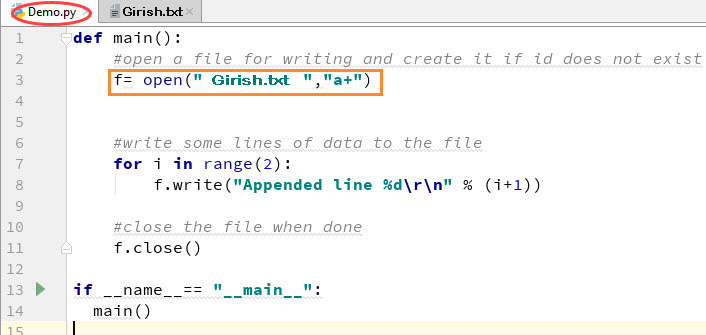
I first want to show you the ‘old fashioned way of doing things. Luckily, Python has the ‘with’ statement to help us out. For these reasons, it’s a good habit to close a file when you’re done with it.Ĭlosing a file used to be a manual task, that can be forgotten easily. Also, when you open a lot of files, each open file takes up memory and other resources. This way, you make sure other software can access the file safely. With such software, you need to be more careful and close the resources you don’t need anymore.

That’s nice and considerate of the Python devs, but a lot of software keeps running indefinitely. When your program exits, all resources are freed up automatically. This doesn’t have to be a problem though. If you do this often enough, you might run into problems, because there’s a limit to the number of open files a regular operating system user can have. If you don’t, you create a so-called resource leak. If the file was successfully opened, it returns a file object that you can use to read from and write to that file.Īs soon as you open a file with Python, you are using system resources that you need to free once you’re done. The open() function expects at least one argument: the file name. It’s part of Python’s built-in functions, you don’t need to import anything to use open(). In Python, we open a file with the open() function.
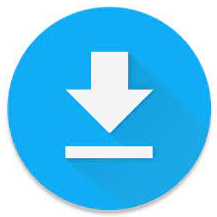